C++ examples
This example executes the examples written with C++ API.
[1]:
import lmenv
env = lmenv.load('.lmenv')
[2]:
import os
import sys
import subprocess as sp
import numpy as np
import imageio
%matplotlib inline
import matplotlib.pyplot as plt
[3]:
outdir = './output'
width = 1920
height = 1080
[4]:
def run_example(ex, params):
print('Executing example [name=%s]' % ex)
sys.stdout.flush()
# Execute the example executable
# We convert backslashes as path separator in Windows environment
# to slashes because subprocess might pass unescaped backslash.
sp.call([
os.path.join(env.bin_path, ex)
] + [str(v).replace('\\', '/') for v in params])
def visualize(out):
img = imageio.imread(out)
f = plt.figure(figsize=(15,15))
ax = f.add_subplot(111)
ax.imshow(np.clip(np.power(img,1/2.2),0,1), origin='lower')
plt.show()
pt.cpp
Corresponding Python example: Rendering with path tracing
[5]:
out = os.path.join(outdir, 'pt.pfm')
run_example('pt', [
os.path.join(env.scene_path, 'fireplace_room/fireplace_room.obj'),
out,
5, 20,
width, height,
5.101118, 1.083746, -2.756308,
4.167568, 1.078925, -2.397892,
43.001194
])
visualize(out)
Executing example [name=pt]
[I|0.000|92@user ] Lightmetrica -- Version 3.0.0 (rev. 70601db) Linux x64
[I|0.000|46@assetgr] Loading asset [name='film1']
[I|0.076|46@assetgr] Loading asset [name='camera1']
[I|0.076|46@assetgr] Loading asset [name='obj1']
[I|0.076|29@objload] .. Loading OBJ file [path='fireplace_room.obj']
[I|0.076|234@objloa] .. Loading MTL file [path='fireplace_room.mtl']
[I|0.398|52@texture] .. Loading texture [path='wood.ppm']
[I|0.490|52@texture] .. Loading texture [path='leaf.ppm']
[I|0.493|52@texture] .. Loading texture [path='picture8.ppm']
[I|0.526|52@texture] .. Loading texture [path='wood5.ppm']
[I|0.579|46@assetgr] Loading asset [name='accel']
[I|0.579|46@assetgr] Loading asset [name='scene']
[I|0.584|314@scene ] Building acceleration structure [name='accel']
[I|0.584|129@accel_] .. Flattening scene
[I|0.614|259@accel_] .. Building
[I|1.236|46@assetgr] Loading asset [name='renderer']
[I|257.048|160@film_b] Saving image [file='./output/pt.pfm']
[I|257.048|166@film_b] .. Creating directory [path='./output']
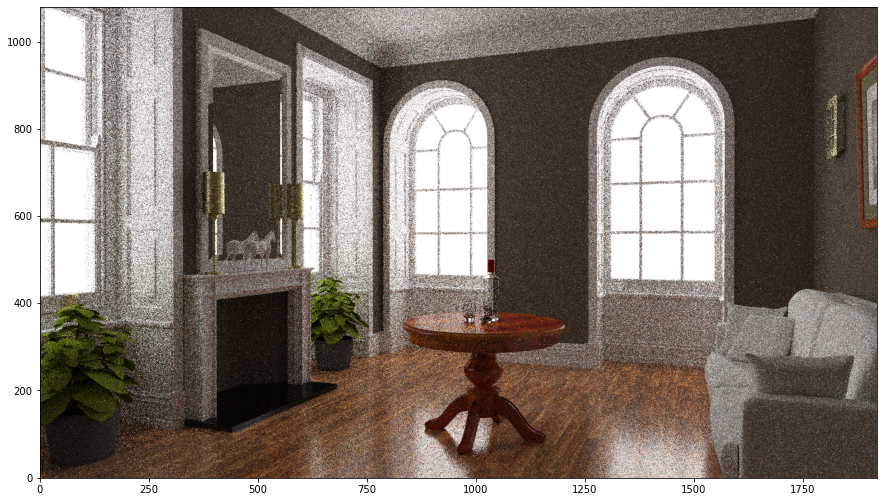
[6]:
out = os.path.join(outdir, 'pt2.pfm')
run_example('pt', [
os.path.join(env.scene_path, 'cornell_box/CornellBox-Sphere.obj'),
out,
5, 20,
width, height,
0, 1, 5,
0, 1, 0,
30
])
visualize(out)
Executing example [name=pt]
[I|0.000|92@user ] Lightmetrica -- Version 3.0.0 (rev. 70601db) Linux x64
[I|0.000|46@assetgr] Loading asset [name='film1']
[I|0.077|46@assetgr] Loading asset [name='camera1']
[I|0.077|46@assetgr] Loading asset [name='obj1']
[I|0.077|29@objload] .. Loading OBJ file [path='CornellBox-Sphere.obj']
[I|0.077|234@objloa] .. Loading MTL file [path='CornellBox-Sphere.mtl']
[I|0.081|46@assetgr] Loading asset [name='accel']
[I|0.082|46@assetgr] Loading asset [name='scene']
[I|0.082|314@scene ] Building acceleration structure [name='accel']
[I|0.082|129@accel_] .. Flattening scene
[I|0.083|259@accel_] .. Building
[I|0.090|46@assetgr] Loading asset [name='renderer']
[I|33.737|160@film_b] Saving image [file='./output/pt2.pfm']
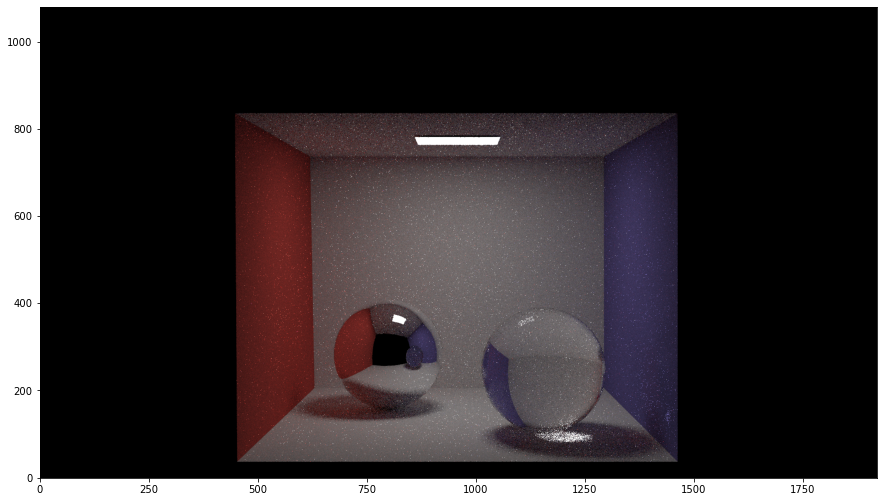
custom_renderer.cpp
Corresponding Python example: Rendering with custom renderer
[7]:
out = os.path.join(outdir, 'custom_renderer.pfm')
run_example('custom_renderer', [
os.path.join(env.scene_path, 'fireplace_room/fireplace_room.obj'),
out,
5,
width, height,
5.101118, 1.083746, -2.756308,
4.167568, 1.078925, -2.397892,
43.001194
])
visualize(out)
Executing example [name=custom_renderer]
[I|0.000|92@user ] Lightmetrica -- Version 3.0.0 (rev. 70601db) Linux x64
[I|0.000|46@assetgr] Loading asset [name='film1']
[I|0.077|46@assetgr] Loading asset [name='camera1']
[I|0.077|46@assetgr] Loading asset [name='obj1']
[I|0.077|29@objload] .. Loading OBJ file [path='fireplace_room.obj']
[I|0.077|234@objloa] .. Loading MTL file [path='fireplace_room.mtl']
[I|0.399|52@texture] .. Loading texture [path='wood.ppm']
[I|0.490|52@texture] .. Loading texture [path='leaf.ppm']
[I|0.493|52@texture] .. Loading texture [path='picture8.ppm']
[I|0.526|52@texture] .. Loading texture [path='wood5.ppm']
[I|0.579|46@assetgr] Loading asset [name='accel']
[I|0.579|46@assetgr] Loading asset [name='scene']
[I|0.585|314@scene ] Building acceleration structure [name='accel']
[I|0.585|129@accel_] .. Flattening scene
[I|0.615|259@accel_] .. Building
[I|1.234|46@assetgr] Loading asset [name='renderer']
[I|7.341|160@film_b] Saving image [file='./output/custom_renderer.pfm']
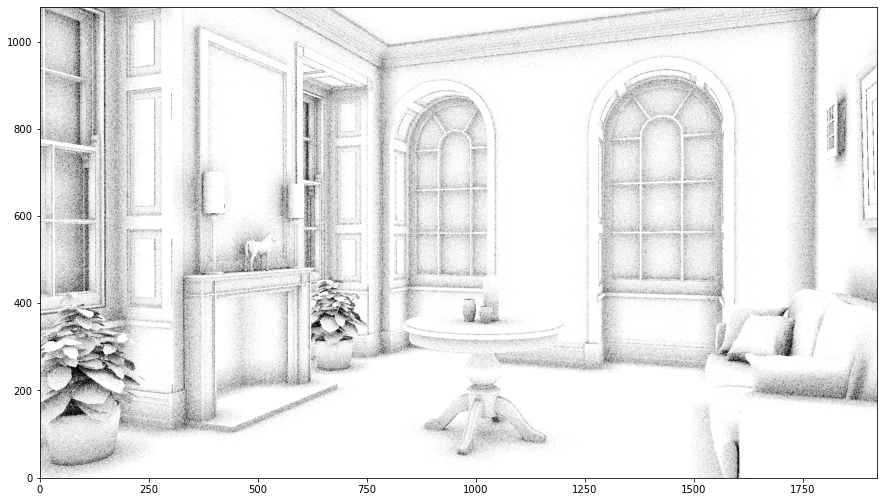