Rendering with path tracing
This example describes how to render the scene with path tracing. Path tracing is a rendering technique based on Monte Carlo method and notably one of the most basic (yet practical) rendering algorithms taking global illumination into account. Our framework implements path tracing as renderer::pt
renderer.
The use of the renderer is straightforward; we just need to create renderer::pt
renderer and call lm::Renderer::render()
function with some renderer-specific parameters. Thanks to the modular design of the framework, the most of the code can be the same as Raycasting a scene with OBJ models.
[1]:
import lmenv
env = lmenv.load('.lmenv')
[2]:
import os
import numpy as np
import imageio
%matplotlib inline
import matplotlib.pyplot as plt
import lightmetrica as lm
%load_ext lightmetrica_jupyter
[3]:
lm.init()
lm.log.init('jupyter')
lm.progress.init('jupyter')
lm.info()
[I|0.000] Lightmetrica -- Version 3.0.0 (rev. 70601db) Linux x64
[4]:
# Film for the rendered image
film = lm.load_film('film', 'bitmap', w=1920, h=1080)
# Pinhole camera
camera = lm.load_camera('camera1', 'pinhole',
position=[5.101118, 1.083746, -2.756308],
center=[4.167568, 1.078925, -2.397892],
up=[0,1,0],
vfov=43.001194,
aspect=16/9)
# OBJ model
model = lm.load_model('model', 'wavefrontobj',
path=os.path.join(env.scene_path, 'fireplace_room/fireplace_room.obj'))
[I|0.009] Loading asset [name='film']
[I|0.087] Loading asset [name='camera1']
[I|0.087] Loading asset [name='model']
[I|0.087] .. Loading OBJ file [path='fireplace_room.obj']
[I|0.087] .. Loading MTL file [path='fireplace_room.mtl']
[I|0.416] .. Loading texture [path='wood.ppm']
[I|0.507] .. Loading texture [path='leaf.ppm']
[I|0.509] .. Loading texture [path='picture8.ppm']
[I|0.541] .. Loading texture [path='wood5.ppm']
[5]:
accel = lm.load_accel('accel', 'sahbvh')
scene = lm.load_scene('scene', 'default', accel=accel)
scene.add_primitive(camera=camera)
scene.add_primitive(model=model)
scene.build()
[I|0.600] Loading asset [name='accel']
[I|0.601] Loading asset [name='scene']
[I|0.604] Building acceleration structure [name='accel']
[I|0.604] .. Flattening scene
[I|0.632] .. Building
[6]:
renderer = lm.load_renderer('renderer', 'pt',
scene=scene,
output=film,
scheduler='sample',
spp=5,
max_verts=20)
renderer.render()
[I|1.278] Loading asset [name='renderer']
[6]:
{'elapsed': 217.858951102, 'processed': 5}
[7]:
img = np.copy(film.buffer())
f = plt.figure(figsize=(15,15))
ax = f.add_subplot(111)
ax.imshow(np.clip(np.power(img,1/2.2),0,1), origin='lower')
plt.show()
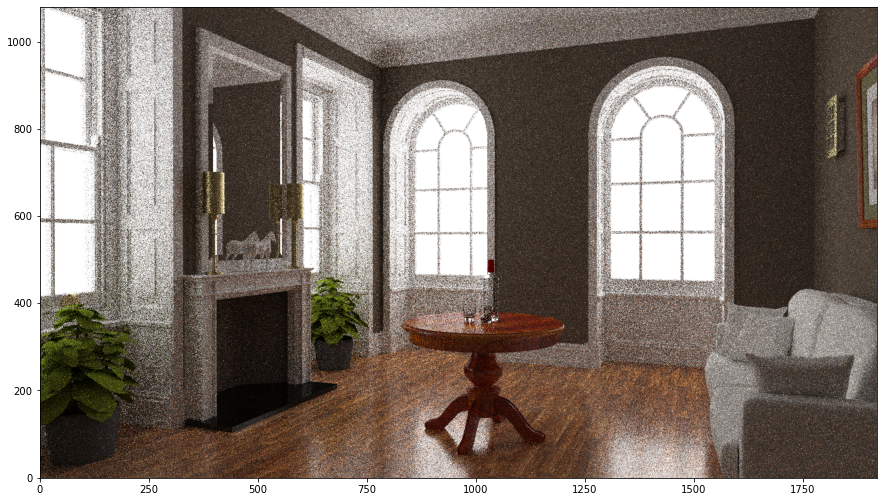